Bienvenido al plugin NPC Builder para Unity, diseñado para agilizar la integración e interacción de personajes no jugables (NPCs) dentro de tus proyectos Unity. Esta guía te guiará a través de la configuración y el uso del plugin de principio a fin.
Requisitos previos #
- Unity 5.3 o superior. (Unity 2020.2 para utilizar el ejemplo – porque, el uso del sistema de entrada).
- Conocimientos básicos de Unity y C#.
Instalación #
- Descarga el paquete NPC Builder Plugin de Unity Asset Store (actualmente en proceso de publicación).
- En Unity, vaya a
Assets -> Import Package -> Custom Package
y seleccione el archivo descargado. - Asegúrate de que todos los archivos están seleccionados y haz clic en ‘Importar’.
Configuración de credenciales #
Antes de usar el plugin, necesitas configurar tus credenciales de autenticación. Un archivo config.json
ya se proporciona en la raíz del paquete del plugin NPC Builder dentro de la carpeta Assets
de su proyecto Unity.
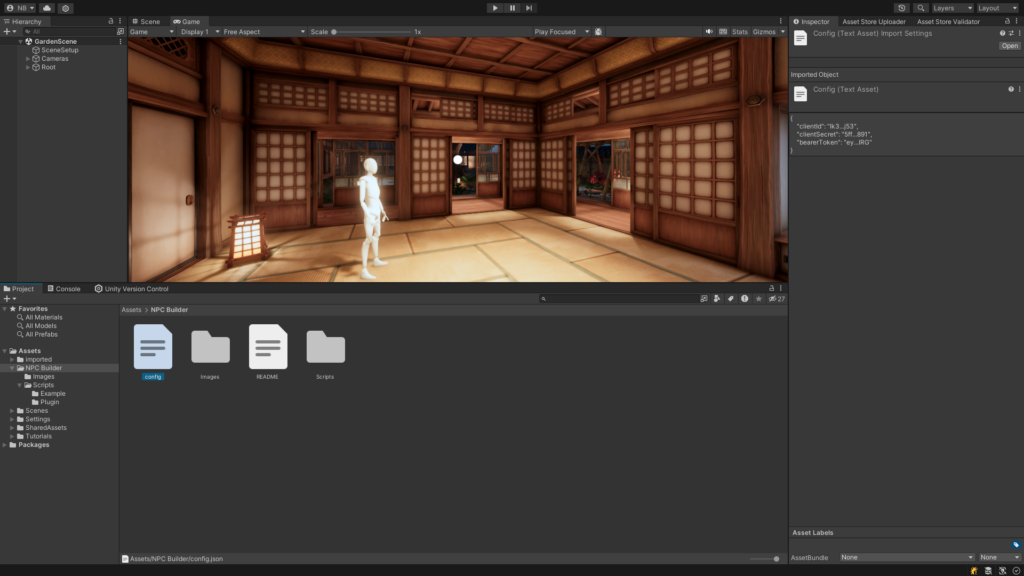
Navega al archivo config.json
ubicado en la raíz del paquete NPC Builder dentro de la carpeta Assets
de tu proyecto Unity.
Abre el archivo config.json
y actualízalo con tus credenciales reales:
{
"clientId": "YOUR_CLIENT_ID",
"clientSecret": "YOUR_CLIENT_SECRET",
"bearerToken": "YOUR_BEARER_TOKEN"
}
Sustituye YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
y YOUR_BEARER_TOKEN
por las credenciales reales proporcionadas por la plataforma NPC Builder.
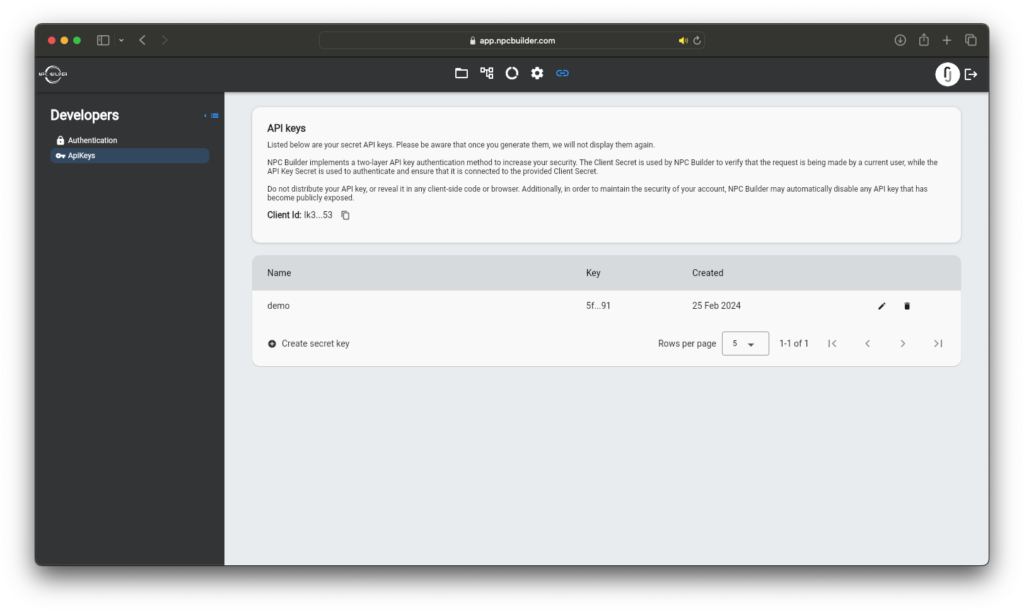
Ten en cuenta lo siguiente:
- El
clientId
y elclientSecret
son necesarios para la configuración inicial y la autenticación con la API de NPC Builder. - El
bearerToken
es un método alternativo de autenticación. Si tienes unclientSecret
, puede que no necesites usar elbearerToken
. Sin embargo, si prefieres utilizar una autenticación basada en token para las llamadas a la API, puedes sustituir el campobearerToken
por tu token real. - Si se proporcionan tanto
clientSecret
comobearerToken
, el plugin dará prioridad al uso declientSecret
para la autenticación. - Asegúrate de que tus credenciales se mantienen seguras y no se exponen en repositorios de código compartidos o públicos.
Uso del plugin #
Adjunta el componente NPCBuilderInteractions
a tu objeto NPC en la escena.
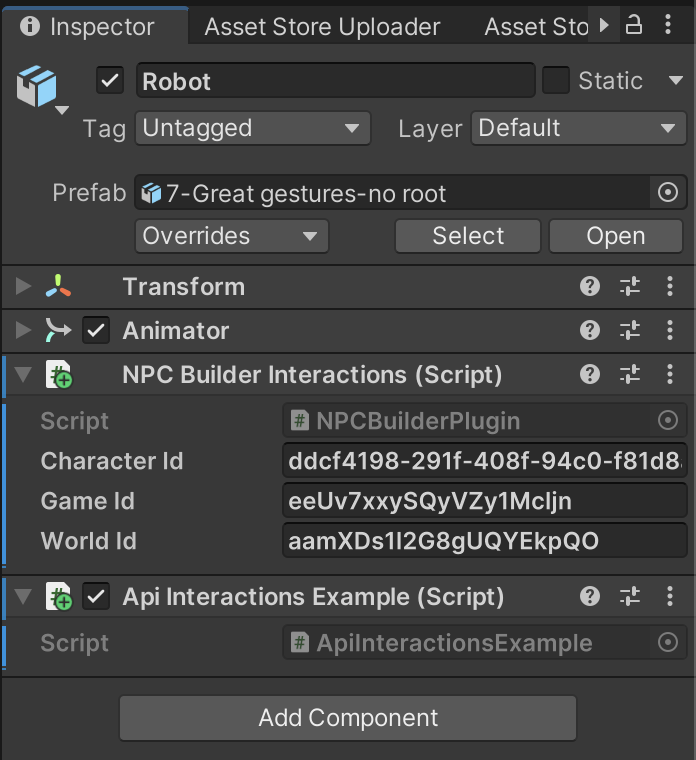
Nota: Obtén los valores de id de la plataforma.
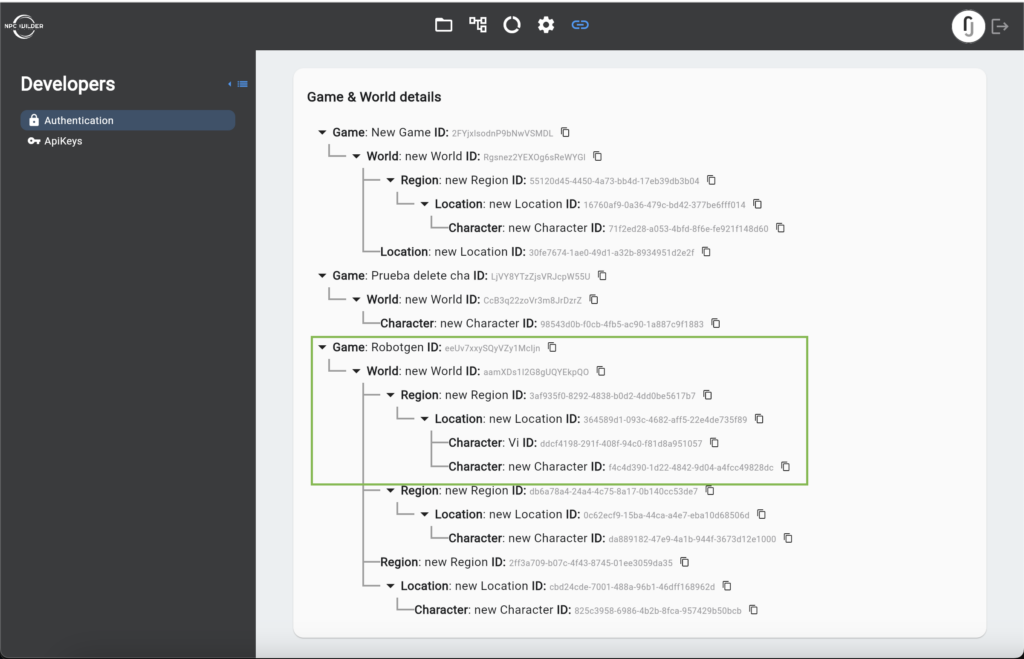
EJEMPLO: Interacción con PNJs #
Utiliza el siguiente script de ejemplo para iniciar interacciones con tus NPCs:
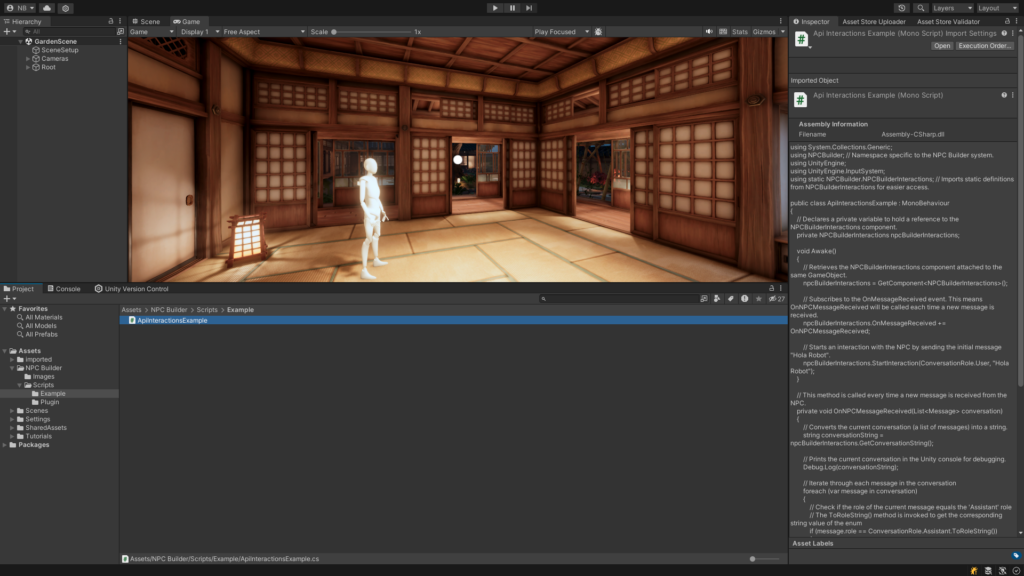
using System.Collections.Generic;
using NPCBuilder; // Namespace specific to the NPC Builder system.
using UnityEngine;
using UnityEngine.InputSystem;
using static NPCBuilder.NPCBuilderInteractions; // Imports static definitions from NPCBuilderInteractions for easier access.
public class ApiInteractionsExample : MonoBehaviour
{
// Declares a private variable to hold a reference to the NPCBuilderInteractions component.
private NPCBuilderInteractions npcBuilderInteractions;
void Awake()
{
// Retrieves the NPCBuilderInteractions component attached to the same GameObject.
npcBuilderInteractions = GetComponent<NPCBuilderInteractions>();
// Subscribes to the OnMessageReceived event. This means OnNPCMessageReceived will be called each time a new message is received.
npcBuilderInteractions.OnMessageReceived += OnNPCMessageReceived;
// Starts an interaction with the NPC by sending the initial message "Hola Robot".
npcBuilderInteractions.StartInteraction(ConversationRole.User, "Hola Robot");
}
// This method is called every time a new message is received from the NPC.
private void OnNPCMessageReceived(List<Message> conversation)
{
// Converts the current conversation (a list of messages) into a string.
string conversationString = npcBuilderInteractions.GetConversationString();
// Prints the current conversation in the Unity console for debugging.
Debug.Log(conversationString);
// Iterate through each message in the conversation
foreach (var message in conversation)
{
// Check if the role of the current message equals the 'Assistant' role
// The ToRoleString() method is invoked to get the corresponding string value of the enum
if (message.role == ConversationRole.Assistant.ToRoleString())
{
// If the message is from the assistant, its content is logged
Debug.Log(" Assistant message: " + message.content);
}
}
}
void Update()
{
// Checks if the 'C' key was pressed.
if (Keyboard.current.cKey.wasPressedThisFrame)
{
// If the 'C' key was pressed, initiates a new interaction with the NPCBuilder Api, sending the message".
npcBuilderInteractions.StartInteraction(ConversationRole.User, "Que quieres?");
}
}
}
Como podemos ver en la imagen inferior, se muestra la conversación devuelta por la API del NPC Builder.
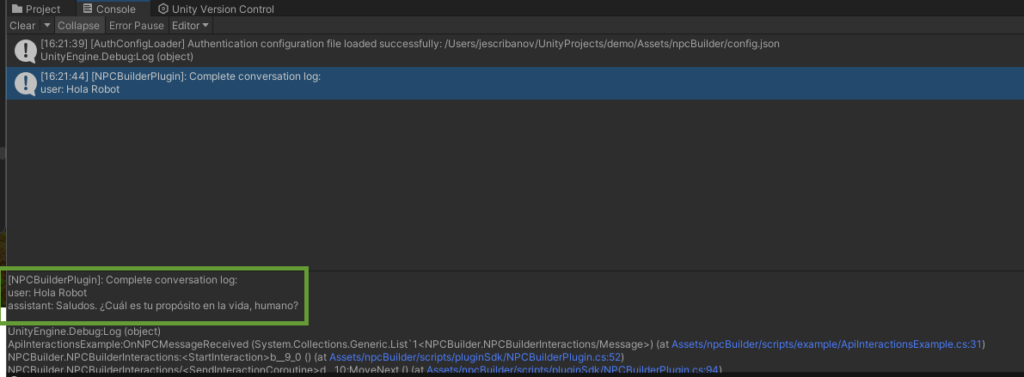
Eventos #
Los eventos te permiten desencadenar comportamientos de los NPC, ya sea como acciones independientes o en combinación con elementos específicos, en función de las interacciones del usuario o de la lógica del juego, lo que facilita las interacciones dinámicas entre los NPC y los jugadores.
Tipos de eventos #
- Action Events: activan acciones que no implican un elemento asociado (por ejemplo, un NPC que sigue al jugador).
- Item Events: incluyen un ítem asociado, como proporcionar un token al jugador.
Configuración de eventos en la plataforma #
Para añadir y configurar eventos o ítems en la plataforma, tenemos dos opciones: crearlos globalmente, permitiendo su reutilización en diferentes personajes, o configurarlos individualmente en las propiedades del personaje.
Añadir eventos o elementos globalmente #
Para añadir eventos o elementos globalmente, ve a Ajustes y añádelos a las colecciones.
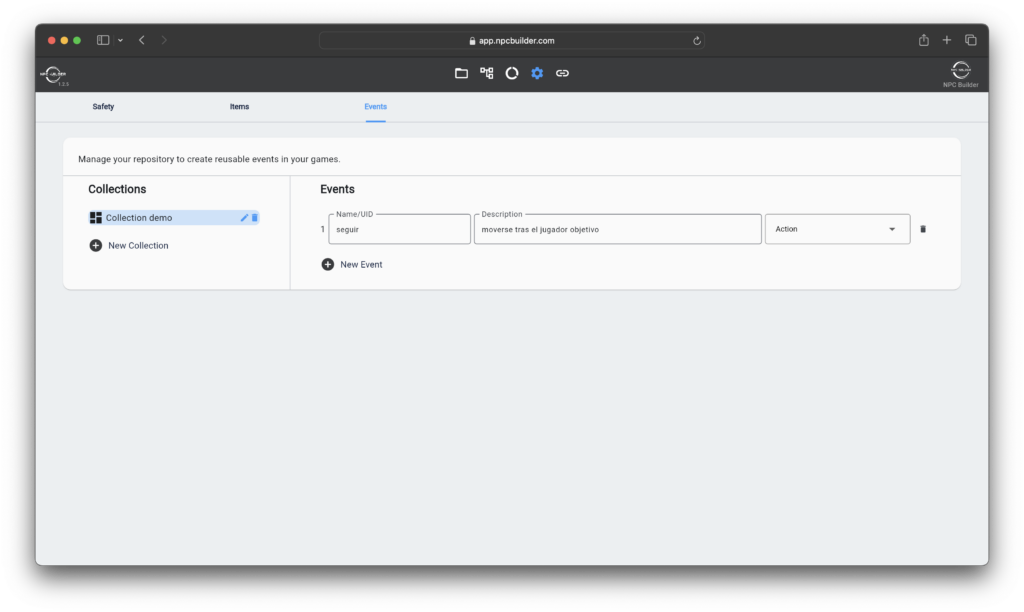
Añadir eventos individualmente #
Para añadir eventos o elementos individualmente en las propiedades del personaje, basta con hacer clic en «New item o new event»
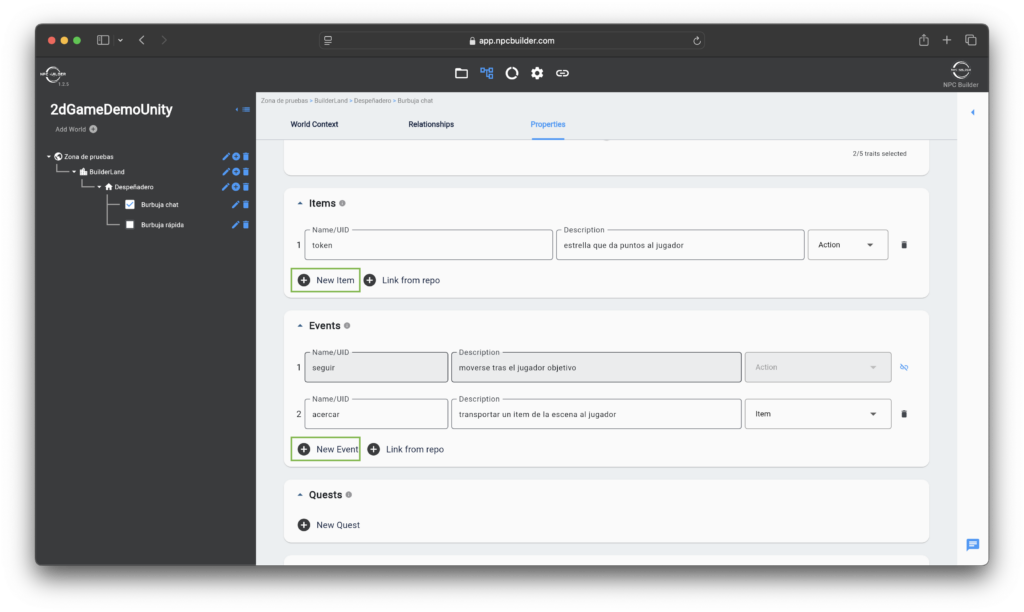
Para enlazar eventos o elementos definidos globalmente, haz clic en «Link from Repo» y añade el elemento o evento correspondiente.
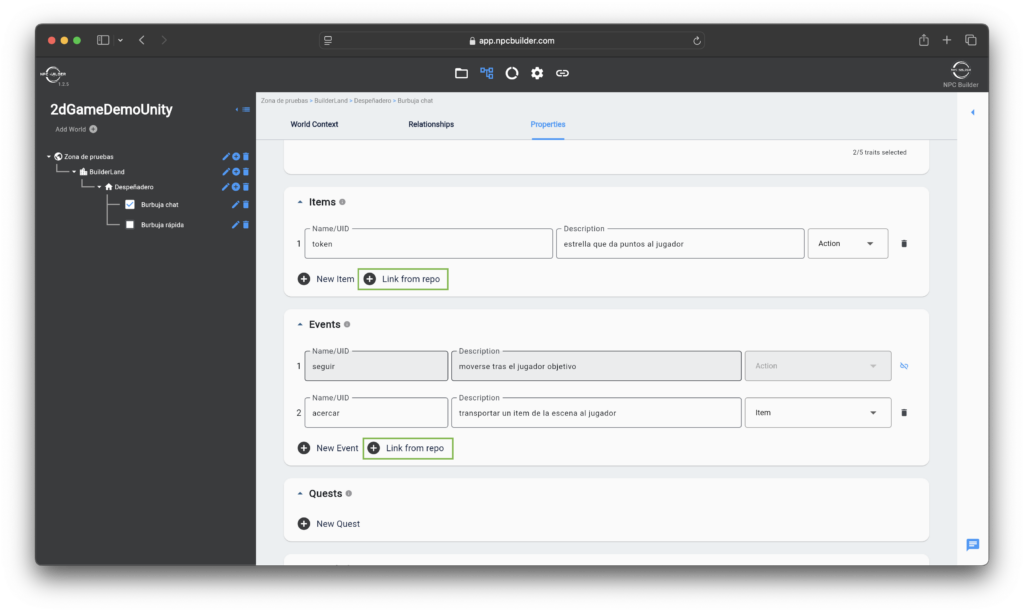
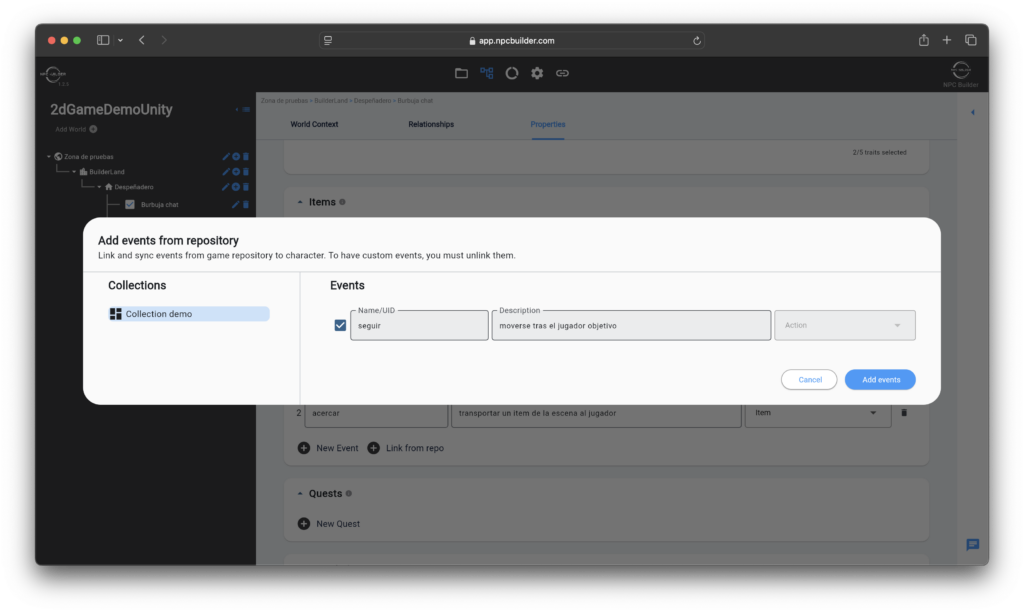
Definición de los componentes de objeto y evento #
Evento #
Representa un evento desencadenado por un NPC o jugador, como recoger una pelota.

- Name/UID: sirve como identificador único para el evento.
- Description: proporciona profundidad para entender la identificación del evento.
- Type:
- Action: especifica una operación independiente, definida por su nombre y descripción.
- Item: vincula la acción a elementos específicos definidos en la sección de propiedades.
Objeto #
Objeto asociado al evento si éste se ha especificado como tipo de elemento.
- Name/UID: sirve como identificador único para el elemento.
- Description: proporciona profundidad para comprender la identificación del elemento.
- Types:
- Trade: artículos destinados al comercio, como los implicados en eventos de compra o trueque.
- Action: objetos que tienen un objetivo o propósito específico dentro de la historia o el juego.

Uso e integración en el Inspector #
Para integrar eventos de usuario o personaje, sólo tenemos que especificar el nombre del evento exactamente como se definió en la tarea y añadir ítems a la misma si procede.
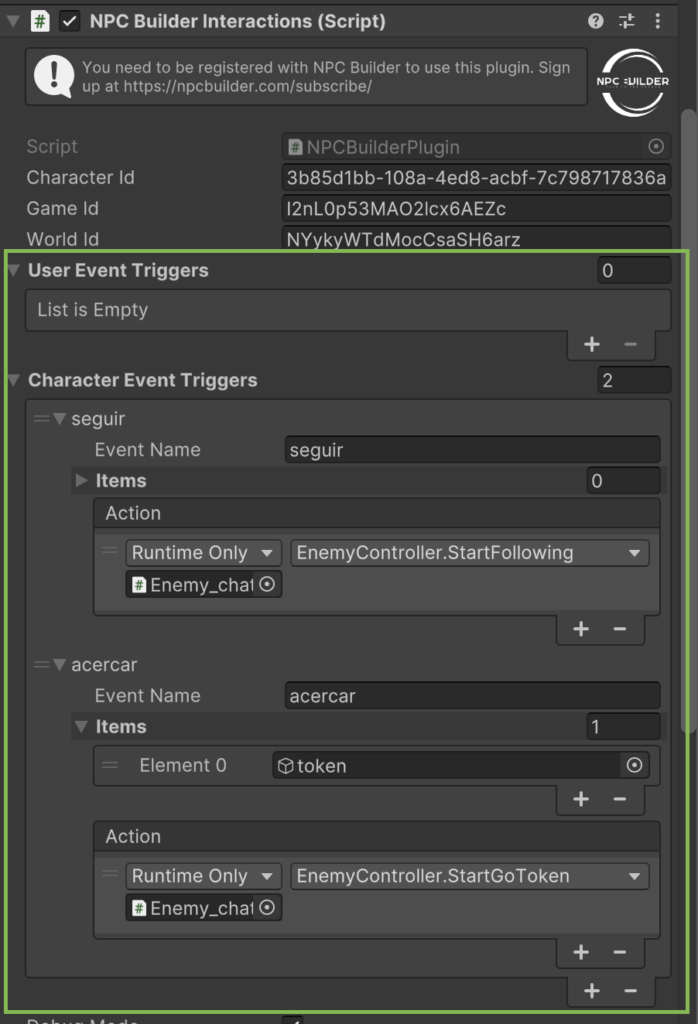
Para ejecutar un evento específico dentro de una función determinada, la función debe estar vinculada en el campo Action del Inspector.
Si un objeto aplica, un GameObject debe ser asignado, el cual será recuperado programáticamente a través del evento. El item será establecido dinámicamente basado en el evento usando el siguiente código:
nPCBuilderInteractions.getGameObjectAssociatedToEvent += (GameObject gameObject) =>
{
if (gameObject != null)
{
item = gameObject;
}
};